Are you tired of sharing long, unwieldy URLs? Do you want to create your own URL shortener without relying on third-party services? Look no further! In this comprehensive guide, we’ll walk you through the process of building a URL Shortener App in Vanilla Javascript. The best part? It’s completely open source, allowing you to customize and expand upon the code to suit your needs.
Demo URL Shortener App
This is the demo URL Shortener App, uploaded to GitHub Pages from my GitHub Profile
https://configuroweb.github.io/url-shortener-app
GitHub Repository
You may also check this other projects
- PHP-Based Comment Functionality: A Comprehensive Guide
- Simple Student Attendance System: A Comprehensive Guide for Educational Institutions
- Launch Countdown App: A Powerful VanillaJS Web Application
Why Build a URL Shortener App in Vanilla Javascript?
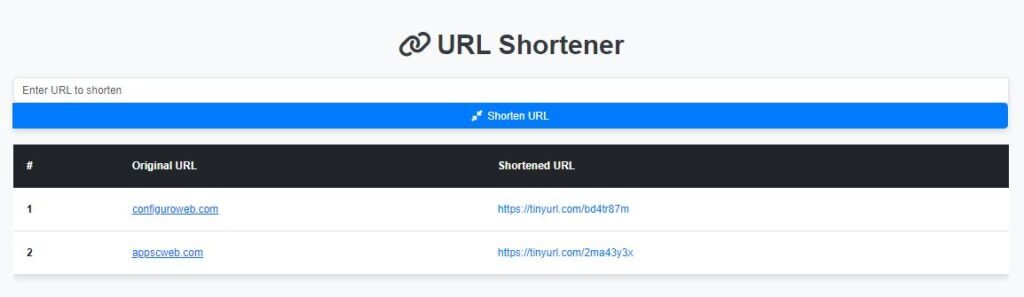
Before we dive into the implementation, let’s discuss why you might want to create a URL Shortener App in Vanilla Javascript:
- Learning Experience: Building a URL shortener is an excellent project for honing your JavaScript skills.
- Customization: By creating your own app, you have full control over its features and functionality.
- Privacy: Unlike third-party services, your personal URL shortener keeps your data under your control.
- Open Source: The code we’ll create is open source, allowing for community contributions and improvements.
Setting Up Your Local Environment
To get started with our URL Shortener App in Vanilla Javascript, you’ll need a basic local server setup. Here’s how to do it:
- Install Node.js from the official website (https://nodejs.org).
- Create a new directory for your project:
mkdir url-shortener-app
- Navigate to the directory:
cd url-shortener-app
- Initialize a new Node.js project:
npm init -y
- Install a simple local server package:
npm install http-server --save-dev
- Add a script to your
package.json
file:
json
"scripts": {
"start": "http-server"
}
Now you’re ready to start building your URL Shortener App in Vanilla Javascript!
HTML Structure
First, let’s create the HTML structure for our app. Create an index.html
file in your project directory:
html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>URL Shortener App in Vanilla Javascript</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>URL Shortener</h1>
<form id="url-form">
<input type="url" id="long-url" placeholder="Enter a long URL" required>
<button type="submit">Shorten URL</button>
</form>
<div id="result" class="hidden">
<h2>Shortened URL:</h2>
<p id="short-url"></p>
<button id="copy-btn">Copy to Clipboard</button>
</div>
</div>
<script src="app.js"></script>
</body>
</html>
CSS Styling
Now, let’s add some basic styling to make our URL Shortener App in Vanilla Javascript look presentable. Create a styles.css
file:
css
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f0f0f0;
}
.container {
background-color: white;
padding: 2rem;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h1 {
text-align: center;
color: #333;
}
form {
display: flex;
margin-bottom: 1rem;
}
input[type="url"] {
flex-grow: 1;
padding: 0.5rem;
font-size: 1rem;
border: 1px solid #ddd;
border-radius: 4px 0 0 4px;
}
button {
padding: 0.5rem 1rem;
font-size: 1rem;
background-color: #007bff;
color: white;
border: none;
border-radius: 0 4px 4px 0;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
.hidden {
display: none;
}
#result {
margin-top: 1rem;
padding: 1rem;
background-color: #e9ecef;
border-radius: 4px;
}
#short-url {
word-break: break-all;
margin-bottom: 0.5rem;
}
#copy-btn {
width: 100%;
margin-top: 0.5rem;
}
JavaScript Implementation
Now for the heart of our URL Shortener App in Vanilla Javascript. Create an app.js
file and add the following code:
javascript
document.addEventListener('DOMContentLoaded', () => {
const form = document.getElementById('url-form');
const longUrlInput = document.getElementById('long-url');
const resultDiv = document.getElementById('result');
const shortUrlP = document.getElementById('short-url');
const copyBtn = document.getElementById('copy-btn');
form.addEventListener('submit', async (e) => {
e.preventDefault();
const longUrl = longUrlInput.value;
const shortUrl = await shortenUrl(longUrl);
displayResult(shortUrl);
});
copyBtn.addEventListener('click', () => {
navigator.clipboard.writeText(shortUrlP.textContent)
.then(() => alert('Copied to clipboard!'))
.catch(err => console.error('Failed to copy: ', err));
});
});
async function shortenUrl(longUrl) {
// In a real implementation, you would send a request to your server here.
// For this example, we'll just generate a random string.
const randomString = Math.random().toString(36).substring(2, 8);
return `http://short.url/${randomString}`;
}
function displayResult(shortUrl) {
shortUrlP.textContent = shortUrl;
resultDiv.classList.remove('hidden');
}
This JavaScript code sets up event listeners, handles form submission, and manages the display of the shortened URL. In a real-world scenario, you would implement server-side logic to generate and store short URLs.
Running Your URL Shortener App
To run your URL Shortener App in Vanilla Javascript, follow these steps:
- Open a terminal in your project directory.
- Run the command:
npm start
- Open a web browser and navigate to
http://localhost:8080
You should now see your URL shortener app running locally!
Expanding Your URL Shortener App
Now that you have a basic URL Shortener App in Vanilla Javascript, here are some ideas to expand its functionality:
- Backend Integration: Implement a Node.js backend to handle URL shortening and storage.
- Database: Use a database like MongoDB to store long and short URL pairs.
- Custom Short URLs: Allow users to create custom short URLs.
- Analytics: Add click tracking and basic analytics for shortened URLs.
- API: Create an API for programmatic access to your URL shortener.
Conclusion
Congratulations! You’ve successfully built a basic URL Shortener App in Vanilla Javascript. This open-source project serves as an excellent foundation for further learning and development. By creating your own URL shortener, you’ve gained valuable experience in front-end development and potentially back-end integration.
Remember, the power of open-source projects lies in collaboration and continuous improvement. Feel free to share your URL Shortener App in Vanilla Javascript with the community, contribute to other open-source projects, and keep expanding your skills.
Happy coding, and may your URLs always be short and sweet!
Leave a Reply